Welcome to the Programming in C MCQs Page
Dive deep into the fascinating world of Programming in C with our comprehensive set of Multiple-Choice Questions (MCQs). This page is dedicated to exploring the fundamental concepts and intricacies of Programming in C, a crucial aspect of UGC CBSE NET Exam. In this section, you will encounter a diverse range of MCQs that cover various aspects of Programming in C, from the basic principles to advanced topics. Each question is thoughtfully crafted to challenge your knowledge and deepen your understanding of this critical subcategory within UGC CBSE NET Exam.
Check out the MCQs below to embark on an enriching journey through Programming in C. Test your knowledge, expand your horizons, and solidify your grasp on this vital area of UGC CBSE NET Exam.
Note: Each MCQ comes with multiple answer choices. Select the most appropriate option and test your understanding of Programming in C. You can click on an option to test your knowledge before viewing the solution for a MCQ. Happy learning!
Programming in C MCQs | Page 6 of 16
Explore more Topics under UGC CBSE NET Exam
The above C statement will
union tag {
int a;
float b;
char c;
};
sum(n)
{
if ( n < 1 ) return n;
else return (n + sum(n–1));
}
main()
{
printf(“%d”, sum(5));
}
while (x!=0)
{
if (x>y)
x = x-y
else
y=y-x;
printf(“%d”,x);
d=0;
for(i=1; i<31, ++i)
for(j=1; j<31, ++j)
for(k=1; k<31, ++k)
if ((i+j+k)%3)= = 0);
d = d + 1;
printf(“%d”, d);
The output will be
(i) int *f( )
(ii) int (*f)( )
Which of the following is true ?
main ( )
Base *p = new Derived;
In what sequence, the constructor will be executed ?
#include< stdio.h >
main( )
{
int a, b = 0;
static int c[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0};
for (a=0; a<10;++a);
if ((c[a]%2)= =0) b+=c[a];
printf(“%d”,b);
}
Suggested Topics
Are you eager to expand your knowledge beyond Programming in C? We've curated a selection of related categories that you might find intriguing.
Click on the categories below to discover a wealth of MCQs and enrich your understanding of Computer Science. Happy exploring!
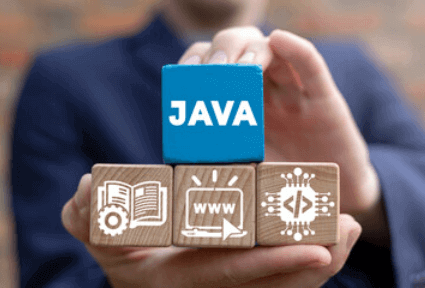
Java Programming
Level up your coding skills with our Java Programming MCQs. From object-oriented...
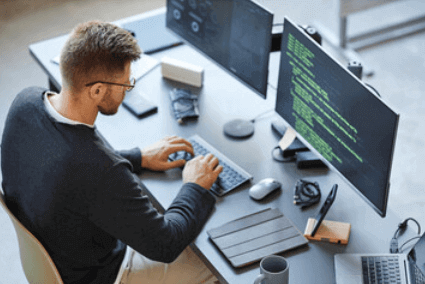
Software Engineering
Learn about the systematic approach to developing software with our Software...